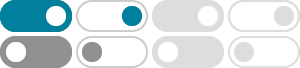
c# - convert vector3 to transform - Stack Overflow
Nov 24, 2016 · You can't directly convert a Vector3 object to a Transform. I found a simple way to do it. First create a spawnPosition - an empty gameobject in your scene. Set transform position to (0,0,0) Add random values to the position. Use transform of …
How to loop through and destroy all children of a game object in …
Sep 22, 2017 · public void ClearChildren() { Debug.Log(transform.childCount); int i = 0; //Array to hold all child obj GameObject[] allChildren = new GameObject[transform.childCount]; //Find all child obj and store to that array foreach (Transform child in transform) { allChildren[i] = child.gameObject; i += 1; } //Now destroy them foreach (GameObject child ...
Copying all the transform values of a gameObject?
Jun 27, 2019 · No, not built-int but I would recommend a proper type [Serializable] public class TransformData { public Vector3 LocalPosition = Vector3.zero; public Vector3 LocalEulerRotation = Vector3.zero; public Vector3 LocalScale = Vector3.one; // Unity requires a default constructor for serialization public TransformData() { } public TransformData(Transform transform) { …
c# - Move simple Object in Unity 2D - Stack Overflow
transform.position = new Vector2(transform.position.x + movespeed * Time.deltaTime, transform.position.y); Time.deltaTime the amount of time it's been between your two frames - This multiplication means no matter how fast or slow the player's computer is, …
c# - Convert a Transform to a RectTransform - Stack Overflow
In my Unity project, I am creating objects dynamically through scripts. var btnExit = new GameObject("Player " + ID + "'s Exit Button"); btnExit.transform.SetParent(UI.transform); I need to set the anchors and pivot of the object. I should be able to do that using its RectTransform component, as I do it when I create objects in the scene editor.
Rotate object in Unity 3D - Stack Overflow
Jan 14, 2022 · transform.rotation = Quaternion.LookRotation(Input.acceleration.normalized, Vector3.up); But i would like to rotate object like for example screen is rotating - 0, 90, 180 and 360 degrees. How can I do it using Unity 3D?
c# - Scale GameObject in Unity - Stack Overflow
Aug 31, 2016 · Position, Rotation & Scale are properties of transform so you need to modify if as follows: public GameObject sprite; public float scale = 2.0f; void ScaleResolution() { sprite.transform.localScale = new Vector3(scale, scale, scale); }
How to add GameObjects positions in a transform list
Jan 8, 2019 · In this first method for accessing position u have to add an extra .transform.position. In this solution you will be able to make a generic Transform/Vector3 list to store the transforms/position, if u need to store the transforms then create a transform list else create a Vector3 list here is the code for it-
Get All children, children of children in Unity3d - Stack Overflow
Jun 21, 2016 · void SetChildLayers (Transform trans, int layer) { //foreach transform, set each transform to layer of layer //also foreach transform, check if that transform has children.
Unity - How to get location of center of parent gameobject
Oct 20, 2018 · The alternative to having the handles there is at the Pivot, which will put the handles at the same location as Transform.position. You can toggle between those two handle display modes in the Unity Editor. So, you want to find the location of the Center handles. That depends on if the parent has a Renderer or not.