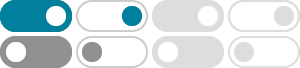
WhatsApp号码被封号,禁止使用怎么办? - 知乎
WhatsApp是许多国家中最受欢迎的聊天应用程序,包括巴西,德国,印度,意大利,荷兰,印度尼西亚,沙特阿拉伯,泰国和土耳其等, 使用起来也很简单,通常只要将客户的手机号保存 …
华为手机不能用WhatsApp了吗? - 知乎
而WhatsApp是依赖于谷歌服务的应用程序之一,因此,华为手机用户无法直接从谷歌应用商店下载和安装WhatsApp。 谷歌服务缺失: 华为手机用户无法获得谷歌的安全认证和更新支持,这 …
WhatsApp被封号?如何申请解封? - 知乎
3种解封WhatsApp账号的方法 如果你的WhatsApp帐号被封锁,你将无法继续使用WhatsApp的聊天功能,并且每次打开应用程序时都会看到“此帐号遭禁止使用WhatsApp”的消息。
手机whatsapp自动翻译? - 知乎
WhatsApp实时翻译功能为企业拓展海外市场提供了强大的支持。 通过WhatsApp实时翻译功能,企业客服团队可以和全球客户进行实时沟通,实时翻译的即时性可以很好地为不同语言的客 …
微信在海外市场是否败给了 WhatsApp,为什么? - 知乎
WhatsApp倒是2009年就发布了 也曾在美国风光过一段时间 但是当时诸如黑莓和诺基亚等上一代品牌还在享受最后的辉煌 人们沟通的模式也依旧是前智能机时代的短信+电话 WhatsApp事实上 …
Whatsapp安卓版怎么下载? - 知乎
WhatsApp有两个版本,一个是WhatsApp,一个WhatsApp business版本。 我将以WhatsApp business版本来讲解,因为这个版本对我们来说多了很多功能,后续会一一铺开来讲解。 使 …
怎样在whatsapp中加好友?-百度经验
May 6, 2019 · 使用WhatsApp的小伙伴们,知不知道如何在该软件中添加好友呢,接下来跟小编一起去看看吧。
Whatsapp安卓版怎么下载? - 知乎
Whatsapp安卓版怎么下载? 怎样才能使用 显示全部 关注者 16
最近遇到whatsapp电脑端无法安装,无法刷新出二维码 ...
当我们将WhatsApp用于客服时,常常需要将WhatsApp多个账号制作成活码,这样可以很方便的实现客户随机分配以及管理客服。以下是将多个WhatsApp账号制作成活码的具体方法和步骤: …
WhatsApp还有什么有什么优势吗相比较微信和QQ? - 知乎
whatsapp的意义何在whatsapp是基于电话通讯录来实现直接聊天的工具性APP,不需要对方验证,只要对方的手机注册了whatsapp,就可以直接添加好友开始聊天。2009年Jan Koum在加利 …