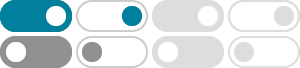
java - Delete Last Node of a Linked List - Stack Overflow
Apr 3, 2015 · The class LinkedList in Java implements the Deque (Double ended queue) interface which supports get/add/remove First/Last methods. An elementary code snippet follows: LinkedList<Integer> list = new LinkedList<Integer>(); list.addFirst(1); list.addLast(2); System.out.println(list.removeLast());
How can I remove the last node of a Linked List in java?
I need to implement two methods removeFirst and removeLast of a LinkedList in Java. The first method i solved it like this: @Override public E removeFirst() { if(isEmpty()){ throw new NoSuchElementException(); } E element = top.next.data; top.next = top.next.next; numElements--; return element; }
Deletion at end (Removal of last node) in a Linked List
Jul 30, 2024 · To perform the deletion operation at the end of linked list, we need to traverse the list to find the second last node, then set its next pointer to null. If the list is empty then there is no node to delete or has only one node then point head to null. Step-by-step approach: Check if list is empty then return NULL.
Removing the Last Node in a Linked List - Baeldung
Jan 11, 2024 · The main challenge for removing the last element from a singly linked list is that we have to update the node that’s second to last. However, our nodes don’t have the references that go back: public static class Node<T> { private T element; private Node<T> next; public Node(T element) { this.element = element; } }
LinkedList removeLast() Method in Java - GeeksforGeeks
Dec 19, 2024 · In Java, the removeLast () method of LinkedList class is used to remove and return the last element of the list. Example 1: Here, we use the removeLast () method to remove and return the last element (tail) of the LinkedList. Return Type: The last element (tail) that is removed from the list.
Remove First and Last Elements from LinkedList in Java
Apr 6, 2023 · The Java.util.LinkedList.removeFirst() method is used to remove the first element from the LinkedList. The Java.util.LinkedList.removeLast() method is used to remove the last element from the LinkedList.
Java LinkedList removeLast() Method - W3Schools
The removeLast() method removes the last item in a list. Tip: Use the removeFirst() method to remove the first item in a list.
Java: Remove first and last element from a linked list - w3resource
Mar 12, 2025 · Write a Java program to remove the first and last elements from a linked list and then print the modified list. Write a Java program to remove the head and tail of a linked list using the poll () and pollLast () methods.
Java Linked List: Efficiently Remove the Last Element
Learn how to efficiently remove the last element from a Java Linked List with step-by-step examples and insights. Perfect for all levels!
java - LinkedList: remove an object - Stack Overflow
Feb 26, 2010 · Compare the code to remove the last entry called by the iterator remove (formatting Sun's): if (e == header) throw new NoSuchElementException(); E result = e.element; e.previous.next = e.next; e.next.previous = e.previous; e.next = …