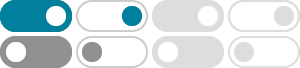
Recursion binary search in Python - Stack Overflow
return binary_search_recursive(list_of_numbers, number, start, mid - 1) # number > list_of_numbers[mid] # Number lies in the upper half. So we call the function again changing the start value to 'mid + 1' Here we are entering the recursive mode. return binary_search_recursive(list_of_numbers, number, mid + 1, end)
Recursive binary search python - Stack Overflow
Jul 9, 2018 · Recursive binary search python. Ask Question Asked 6 years, 9 months ago. Modified 2 years, 6 months ago. ...
python - Binary Search Using a Recursive Function - Stack Overflow
Dec 2, 2022 · Complete the recursive function binary_search() with the following specifications: Parameters: a list of integers a target integer lower and upper bounds within which the recursive call will search Return value: if found, the index within the list where the target is located -1 if target is not found The algorithm begins by choosing an index ...
Recursive binary search in Python - Code Review Stack Exchange
Sep 4, 2015 · The whole point of binary search is that it's O(lg N). If you're going to check that the array is sorted first, you may as well do a linear search. Not to mention that you're doing even worse here since you're checking that the array is sorted on every recursive call, so now we have an O(N lg N) search. Binary search should assume a sorted list ...
Binary search (bisection) in Python - Stack Overflow
Oct 17, 2008 · def binary_search(values, key, lo=0, hi=None, length=None, cmp=None): """ This is a binary search function which search for given key in values. This is very generic since values and key can be of different type. If they are of different type then caller must specify `cmp` function to perform a comparison between key and values' item.
Binary Search with Python through recursion - Stack Overflow
Oct 26, 2022 · In binary search the goal is to return the index of the target in the array. So right off the bat you are making a mistake by splitting the array into halves because any index you find in the half won't necessarily be the same index to the original array plus making copies of arrays is not cheap and binary search is supposed to be efficient.
recursion - Recursive binary search in Python - Stack Overflow
Jan 8, 2015 · Recursive binary search in Python. Ask Question Asked 10 years, 1 month ago. Modified 10 years, 1 month ago.
Binary search algorithm in python - Stack Overflow
Feb 29, 2012 · I am trying to implement the binary search in python and have written it as follows. However, I can't make it stop whenever needle_element is larger than the largest element in the array.
python - Binary Search recursive implementation - Stack Overflow
Jul 30, 2013 · wiki says: a binary search or half-interval search algorithm finds the position of a specified input value (the search "key") within an array sorted by key value.[1][2] In each step, the algorithm compares the search key value with the key value of the middle element of the array.
Binary Search tree recursive implementation in python
It is a feature introduced on python 2.7, so it is kind of a bad practice not to include it. Check this for further information. Bonus : You can check if your insertion algorithms is correct by performing an in-order transversal, after that, the elements should …