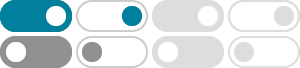
How to read a .xlsx file using the pandas Library in iPython?
The following worked for me: from pandas import read_excel my_sheet = 'Sheet1' # change it to your sheet name, you can find your sheet name at the bottom left of your excel file file_name = 'products_and_categories.xlsx' # change it to the name of your excel file df = read_excel(file_name, sheet_name = my_sheet) print(df.head()) # shows headers with top 5 rows
Reading an Excel file in python using pandas - Stack Overflow
Jun 12, 2013 · Here is an updated method with syntax that is more common in python code. It also prevents you from opening the same file multiple times. import pandas as pd sheet1, sheet2 = None, None with pd.ExcelFile("PATH\FileName.xlsx") as reader: sheet1 = pd.read_excel(reader, sheet_name='Sheet1') sheet2 = pd.read_excel(reader, …
excel - Reading .xlsx format in python - Stack Overflow
Dec 11, 2011 · I've got to read .xlsx file every 10min in python. What is the most efficient way to do this? I've tried using xlrd, but it doesn't read .xlsx - according to documentation he does, but I can't do this - getting Unsupported format, or corrupt file exceptions. What is the best way to read xlsx? I need to read comments in cells too.
Reading/parsing Excel (xls) files with Python - Stack Overflow
May 31, 2010 · What is the best way to read Excel (XLS) files with Python (not CSV files). Is there a built-in package which is supported by default in Python to do this task?
python - Using Pandas to pd.read_excel() for multiple (but not all ...
pd.read_excel('filename.xlsx', sheet_name = 'sheetname') read the specific sheet of workbook and . pd.read_excel('filename.xlsx', sheet_name = None) read all the worksheets from excel to pandas dataframe as a type of OrderedDict means nested dataframes, all the worksheets as dataframes collected inside dataframe and it's type is OrderedDict.
how to open xlsx file with python 3 - Stack Overflow
May 25, 2016 · import xlwings as xw import pandas as pd wb_path = ".\\***.xlsx" # the .xlsx file is in the same directory as the .py script ws_name = "***" # the name of the worksheet to load data wb = xw.Book(wb_path) ws = wb.sheets[ws_name] MAX_ROW = 100 # the rows to read, this can be larger, the empty rows can be dropped in Pandas dataframe MAX_COL = 40 ...
python - Faster way to read Excel files to pandas dataframe - Stack ...
Feb 27, 2015 · As others have suggested, csv reading is faster. So if you are on windows and have Excel, you could call a vbscript to convert the Excel to csv and then read the csv.
How can I open an Excel file in Python? - Stack Overflow
Aug 4, 2012 · You can pass the sheet name as a parameter to pandas.read_excel(): file_name = # path to file + file name sheet = # sheet name or sheet number or list of sheet numbers and names df = pd.read_excel(file_name, sheet_name=sheet) print(df.head()) # print …
how to read certain columns from Excel using Pandas - Python
Unfortunately these methods still seem to read and convert the headers before returning the subselection. I have an Excel sheet with duplicate header names because the sheet contains several similar tables. I want to read those tables individually, so I would want to apply usecols. However, this still add suffixes to the duplicate column names.
python - Reading xlsx file using jupyter notebook - Stack Overflow
Oct 6, 2017 · import pandas as pd df = pd.read_excel('file_name.xlsx', 'Sheet1') df *you must import your .xlsx file into the Jupyter notebook file... *you may also import it into a Github repository and get the raw file then just copy and paste it into where it says 'file_name.xlsx'