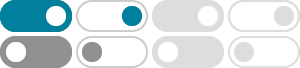
Getting random numbers in Java - Stack Overflow
May 5, 2011 · The first solution is to use the java.util.Random class: import java.util.Random; Random rand = new Random(); // Obtain a number between [0 - 49]. int n = rand.nextInt(50); // Add 1 to the result to get a number from the required range // (i.e., [1 - 50]). n += 1; Another solution is using Math.random(): double random = Math.random() * 49 + 1 ...
How do I generate random integers within a specific range in Java ...
To generate a random number "in between two numbers", use the following code: Random r = new Random(); int lowerBound = 1; int upperBound = 11; int result = r.nextInt(upperBound-lowerBound) + lowerBound; This gives you a random number in between 1 (inclusive) and 11 (exclusive), so initialize the upperBound value by adding 1.
Generating Unique Random Numbers in Java - Stack Overflow
Jun 18, 2016 · Generate random number between 1 to 100 as position and return array[position-1] to get the value . Once you use a number in array, mark the value as -1 ( No need to maintain another array to check if this number is already used) If value in array is -1, get the random number again to fetch new location in array.
Generating a Random Number between 1 and 10 Java
import java.util.Random; If you want to test it out try something like this. Random rn = new Random(); for(int i =0; i < 100; i++) { int answer = rn.nextInt(10) + 1; System.out.println(answer); } Also if you change the number in parenthesis it will create a random number from 0 to that number -1 (unless you add one of course like you have then ...
Java random numbers using a seed - Stack Overflow
Apr 28, 2018 · Pseudorandom number generator. A pseudorandom number generator (PRNG), also known as a deterministic random bit generator DRBG, is an algorithm for generating a sequence of numbers that approximates the properties of random numbers.
True random generation in Java - Stack Overflow
The NSA and Intel’s Hardware Random Number Generator. To make things easier for developers and help generate secure random numbers, Intel chips include a hardware-based random number generator known as RdRand. This chip uses an entropy source on the processor and provides random numbers to software when the software requests them.
Java random number with given length - Stack Overflow
To generate a 6-digit number: Use Random and nextInt as follows: Random rnd = new Random(); int n = 100000 + rnd.nextInt(900000); Note that n will never be 7 digits (1000000) since nextInt(900000) can at most return 899999. So how do I randomize the last 5 chars that can be either A-Z or 0-9? Here's a simple solution:
How to generate 6 different random numbers in java
Mar 24, 2014 · generate a random number from the full range; put that number into your output array; "cross out" the corresponding index in the boolean[]; now generate another random number, but curtail the range by one (now 48); instead of directly using that number as output, scan your boolean[], counting all the non-crossed entries. Stop when you reach the ...
java - How to generate a random alpha-numeric string - Stack …
Sep 3, 2008 · To get a random character containing all digits 0-9 and characters a-z, we would need a random number between 0 and 35 to get one of each character. BigInteger provides a constructor to generate a random number, uniformly distributed over the range 0 to (2^numBits - 1). Unfortunately 35 is not a number which can be received by 2^numBits - 1.
java - What is the best way to generate a random float value …
For a random value within a range, the formula is: double random = min + Math.random() * (max - min); This basic formula is constant no matter what you use to generate random numbers. Math.random() provides moderately well distributed numbers, but you can replace it with whatever random number generator you want, for example (slightly better):