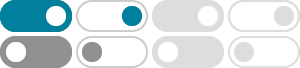
Converting Alphabet Letters to Numbers Using Python
Feb 13, 2023 · This process, known as letter-to-number mapping, involves assigning a numerical value to each letter of the alphabet. Python can accomplish this through various methods, including dictionaries, ASCII values, and built-in functions.
Convert alphabet letters to number in Python - Stack Overflow
Dec 31, 2010 · You can use chr() and ord() to convert betweeen letters and int numbers. Here is a simple example. >>> chr(97) 'a' >>> ord('a') 97
How to Convert Letters to Numbers in Python? - Python Guides
Jan 15, 2025 · "Learn how to convert letters to numbers in Python using ASCII values, ord(), and custom mappings. Step-by-step tutorial with clear examples for easy implementation."
Convert Letters to Numbers and vice versa in Python
Apr 10, 2024 · To convert all of the letters in a string to numbers: Use a list comprehension to iterate over the string. Use the ord() function to get the Unicode code point of each character.
How to Convert Letter to Number in Python - Delft Stack
Mar 11, 2025 · In this tutorial, we will explore different methods to convert letters to their corresponding numerical values in Python, including the use of the built-in ord() function and some creative solutions. Let’s dive in and discover how to make these conversions seamlessly!
Converting Alphabet Letters to Numbers in Python 3
In Python, converting alphabet letters to numbers can be done using the ord() function, which returns the ASCII value of a character. By subtracting 64 from the ASCII value of uppercase letters, we can obtain their corresponding number.
python - Faster way to assign numerical value to letters
Dec 19, 2013 · Well, the best advice for this problem is to create a dictionary associating letter to numbers: d = {'A':1, 'B':2 ... } and changing your if else nightmare with this: tempSum += d[letter]
python - Convert letter into numeric value - Stack Overflow
Mar 23, 2022 · I have column in Pandas dataframe which has values from A-Z. I want to replace the letter value into numeric value. i.e A = 1, B = 2 etc. I tried below and it works but is there an efficient way to replace the value to numeric?
python - Get the Sum of the Values of Letters in a Name - Stack Overflow
Oct 25, 2022 · Write a function called get_numeric_value that takes a sequence of characters (a string) as a parameter. It should return the numeric value of that string. You can use to_numerical in your solution.
python - How do I convert letters to their corresponding number value ...
May 20, 2020 · You can use a one-liner to make a dict from letter to number: import string letter_to_number = {l:n+1 for (l,n) in zip(string.ascii_uppercase, range(len(string.ascii_uppercase)))} # {'A': 1, 'B': 2, 'C': 3, 'D': 4, 'E': 5, 'F': 6, 'G': 7, 'H': 8, 'I': 9, 'J': 10, 'K': 11, 'L': 12, 'M': 13, 'N': 14, 'O': 15, 'P': 16, 'Q': 17, 'R': 18, 'S': 19 ...