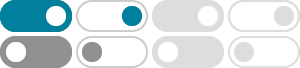
python - for or while loop to do something n times - Stack Overflow
Jul 15, 2013 · break - terminates the loop; continue - moves on to the next time around the loop without executing following code this time around; else - is executed if the loop completed without any break statements being executed. N.B. In the now unsupported Python 2 range produced a list of integers but you could use xrange to use an iterator.
pythonic way to do something N times without an index variable?
The _ is the same thing as x. However it's a python idiom that's used to indicate an identifier that you don't intend to use. In python these identifiers don't takes memor or allocate space like variables do in other languages. It's easy to forget that. They're just names that point to objects, in this case an integer on each iteration.
How to loop exact N times in Python? - Stack Overflow
Oct 16, 2013 · There is no equivalent construct in python. (Incidentally, even in the Ruby example, there is still a need for a counter! The language simply hides it from you. It is also possible that the interpreter is generating bytecode with those lines repeated multiple times, rather than a loop, but that is unlikely.)
How to repeat a while loop a certain number of times
Jan 13, 2018 · To repeat something for a certain number of times, you may: Use range or xrange. for i in range(n): # do something here Use while. i = 0 while i < n: # do something here i += 1 If the loop variable i is irrelevant, you may use _ instead. for _ in range(n): # do something here _ = 0 while _ < n # do something here _ += 1
python - Iterate a certain number of times without storing the ...
loop = range(NUM_ITERATIONS+1) while loop.pop(): do_stuff() Note, however, that this will not work for an arbitrary list: If the first value in the list (the last one popped) does not evaluate to False, you will get another iteration and an exception …
Execute statement every N iterations in Python - Stack Overflow
Apr 11, 2011 · 1. Human-language declarations for x and n:. let x be the number of iterations that have been examined at any given time. let n be the multiple of iterations upon which your code will executed.
python - Loop over list n times - Stack Overflow
Say you have this: foo = [1,2,3,4,5,6,7,8,9,10] bar = 22 I want to get bar many values from foo, repeating from the start after reaching the end.
python - Repeating elements of a list n times - Stack Overflow
Jun 15, 2014 · For base Python 2.7: from itertools import repeat def expandGrid(**kwargs): # Input is a series of lists as named arguments # output is a dictionary defining each combination, preserving names # # lengths of each input list listLens = [len(e) for e in kwargs.itervalues()] # multiply all list lengths together to get total number of combinations nCombos = reduce((lambda x, y: x * y), listLens ...
python - How to nest a "for loop" n times? - Stack Overflow
Oct 13, 2020 · Making a nested for loop (Python 3.x) 1. How to implement n times nested loops in python? 1.
Python: Append item to list N times - Stack Overflow
This seems like something Python would have a shortcut for. I want to append an item to a list N times, effectively doing this: l = [] x = 0 for i in range(100): l.append(x) It would seem to me that there should be an "optimized" method for that, something like: …