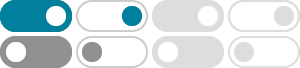
What does colon equal (:=) in Python mean? - Stack Overflow
Mar 21, 2023 · In Python this is simply =. To translate this pseudocode into Python you would need to know the data structures being referenced, and a bit more of the algorithm …
python - What does the caret (^) operator do? - Stack Overflow
Side note, seeing as Python defines this as an xor operation and the method name has "xor" in it, I would consider it a poor design choice to make that method do something not related to xor …
What does the "at" (@) symbol do in Python? - Stack Overflow
Jun 17, 2011 · 96 What does the “at” (@) symbol do in Python? @ symbol is a syntactic sugar python provides to utilize decorator, to paraphrase the question, It's exactly about what does …
What is :: (double colon) in Python when subscripting sequences?
Aug 10, 2010 · I know that I can use something like string[3:4] to get a substring in Python, but what does the 3 mean in somesequence[::3]?
python - Iterating over dictionaries using 'for' loops - Stack Overflow
Jul 21, 2010 · Why is it 'better' to use my_dict.keys() over iterating directly over the dictionary? Iteration over a dictionary is clearly documented as yielding keys. It appears you had Python 2 …
syntax - What do >> and << mean in Python? - Stack Overflow
Apr 3, 2014 · 15 The other case involving print >>obj, "Hello World" is the "print chevron" syntax for the print statement in Python 2 (removed in Python 3, replaced by the file argument of the …
python - SSL: CERTIFICATE_VERIFY_FAILED with Python3 - Stack …
Sep 2, 2017 · Go to the folder where Python is installed, e.g., in my case (Mac OS) it is installed in the Applications folder with the folder name 'Python 3.6'. Now double click on 'Install …
What does -> mean in Python function definitions? - Stack Overflow
Jan 17, 2013 · It's a function annotation. In more detail, Python 2.x has docstrings, which allow you to attach a metadata string to various types of object. This is amazingly handy, so Python …
python - How should I use the Optional type hint? - Stack Overflow
Python 3.10 introduces the | union operator into type hinting, see PEP 604. Instead of Union[str, int] you can write str | int. In line with other type-hinted languages, the preferred (and more …
python - How do I execute a program or call a system command?
How do I call an external command within Python as if I had typed it in a shell or command prompt?