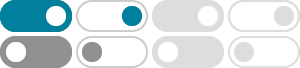
How do you store string type data in a linked list in C?
Dec 1, 2015 · So I'm trying to understand how a linked list works with storing string type pieces of data. As far as I know, a linked list uses a data structure to store data in a somewhat fashionable way so you can easily enter new pieces of data inside, remove them, and rearrange them as …
Convert a String to a Singly Linked List - GeeksforGeeks
Dec 22, 2022 · Given string str, the task is to convert it into a singly Linked List. Examples: Output: A -> B -> C -> D -> A -> B -> C. Input: str = "GEEKS" Output: G -> E -> E -> K -> S. Approach: Below is the implementation of the above approach: // Function to …
c++ linked list storing strings - Stack Overflow
Sep 24, 2013 · I am creating a custom linked list class to store strings from a program I created for an assignment. We were given a linked list handout that works for ints and were told to retool it for string storage, however I am running into an error when trying to run it.
c - How do i store a string inside a node in a linked list? I am ...
Apr 22, 2017 · To make your code simple declare word as a character array in DLNode. say char word [50]. If you know that str is a valid C string and will not be freed anywhere else but in the node memory managing, then you can just do a simple assignment:
Convert LinkedList to String in Java - GeeksforGeeks
Mar 28, 2022 · We can use String class, StringBuilder, or StringBuffer for converting a LinkedList to string. For appending string we can use the “+” operator for the String class and append method for StringBuffer and StringBuilder.
Fixing Linked List Value Changes with Dynamic String Storage in C
Learn how to properly store strings in a linked list in C to prevent previous values from being overwritten. Follow this structured approach to create robust linked list applications.
Singly Linked List (strings only) - Code Review Stack Exchange
This is my attempt at constructing a singly-linked-list with basic functions. I initially tried to build the whole list as a series of nodes, but ran into problems when trying to remove or pop index-0. This time I created a series of Node structs and a single List struct as their head.
Storing strings in a linked list - cboard.cprogramming.com
What's the best way to store the value of a string in a linked list so that I can reuse the original sting without overwriting the existing data? Where's the best place to perform the copy?
Strings in a linked list - C++ Forum
Sep 5, 2012 · Students student02(127802, "Sue", 3.0); // Store the Student objects in the list. list.appendNode(student01); list.appendNode(student02); // Display the values in the list. cout << "Here are the students:\n"; list.displayList(); cout << endl; system("pause"); return 0;
Storing strings dynamically in a linked list - Stack Overflow
I want to create a linked list in C in which the user can input strings which will be stored as nodes in the list. This is my node structure: typdef struct NODE { char word[50]; struct NODE* next; } node;