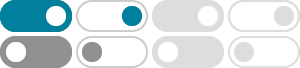
How to assign a string value to a string variable in C++
string s; s = "some string"; Well, actually it's spelled std::string, but if you have a using namespace std; (absolutely evil) or using std::string; (somewhat less evil) before that, it should work - provided that you also have a #include <string> at the top of your file.
C++ String Variable Declaration - Stack Overflow
Here's a short program to demonstrate: string your_name; cout << "Enter your name: "; cin >> your_name; cout << "Hi, " << your_name << "!\n"; return 0;
How can I correctly assign a new string value? - Stack Overflow
You can use the string literals (string constants) to initalize character arrays. This is why. person p = {"John", "Doe",30}; works in the first example. You cannot assign (in the conventional sense) a string in C. The string literals you have ("John") are loaded into …
C++ Strings - W3Schools
Create a variable of type string and assign it a value: string greeting = "Hello"; To use strings, you must include an additional header file in the source code, the <string> library:
std::string::assign() in C++ - GeeksforGeeks
Oct 28, 2020 · In C++, the std::to_string function is used to convert numerical values into the string. It is defined inside <string> header and provides a simple and convenient way to convert numbers of any type to strings. In this article, we will learn how to use std::to_string() in C++. Syntaxstd::to_str
C++ Variables - W3Schools
String values are surrounded by double quotes. To create a variable, specify the type and assign it a value: Where type is one of C++ types (such as int), and variableName is the name of the variable (such as x or myName). The equal sign is used to assign values to the variable.
Strings in C++ - GeeksforGeeks
Mar 6, 2025 · Strings are provided by <string> header file in the form of std::string class. Creating a string means creating an instance of std::string class as shown: where str_name is the name of the string. Initializing means assigning some initial value to the string.
C++ String Variable Declaration Made Simple
Learn to create and manipulate strings effortlessly and effectively. In C++, a string variable can be declared using the `std::string` type, which is part of the Standard Library and allows for dynamic string manipulation. std::string myString = "Hello, World!";
5.7 — Introduction to std::string – Learn C++ - LearnCpp.com
Jan 3, 2025 · The easiest way to work with strings and string objects in C++ is via the std::string type, which lives in the <string> header. We can create objects of type std::string just like other objects: std:: string name {}; // empty string return 0; }
C++ create string of text and variables - Stack Overflow
std::string var = std::string("sometext") + somevar + "sometext" + somevar; Now, the first parameter in the left-to-right list of + operations is a std::string, which has an operator+(const char *). That operator produces a string, which makes the rest of the chain work.