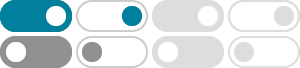
Removing an element from an Array (Java) - Stack Overflow
To allocate a collection (creates a new array), then delete an element (which the collection will do using arraycopy) then call toArray on it (creates a SECOND new array) for every delete brings us to the point where it's not an optimizing issue, it's criminally bad programming. Suppose you had an array taking up, say, 100mb of ram.
How do you delete an element from an array without creating a …
Oct 12, 2019 · There is no direct way to remove elements from an Array in Java. Though Array in Java objects, it doesn't provide any methods to add(), remove() or search an element in Array. This is the reason Collection classes like ArrayList and HashSet are very popular. Though. Thanks to Apache Commons Utils, You can use there ArrayUtils class to remove an ...
java - What is the best way to remove the first element from an …
Feb 13, 2013 · The size of arrays in Java cannot be changed. So, technically you cannot remove any elements from the array. One way to simulate removing an element from the array is to create a new, smaller array, and then copy all of the elements from the original array into the new, smaller array. String[] yourArray = Arrays.copyOfRange(oldArr, 1, oldArr ...
How do I remove objects from an array in Java? - Stack Overflow
Sep 22, 2008 · In the first case create a List from the array, remove the elements, and create a new array from the list. If performance is important iterate over the array assigning any elements that shouldn't be removed to a list, and then create a new array from the list. In the second case simply go through and assign null to the array entries.
java - Delete item from array and shrink array - Stack Overflow
Feb 2, 2011 · If the element you want to remove is the last array item, this becomes easy to implement using Arrays.copy: int a[] = { 1, 2, 3}; a = Arrays.copyOf(a, 2); After running the above code, a will now point to a new array containing only 1, 2. Otherwise if the element you want to delete is not the last one, you need to create a new array at size-1 ...
How can I remove a specific item from an array in JavaScript?
It seems to work well at first, but through a painful process I discovered it fails when trying to remove the second to last element in an array. For example, if you have a 10-element array and you try to remove the 9th element with this: myArray.remove(8); You end …
How to remove and add element from java array - Stack Overflow
Aug 1, 2017 · To remove element from Array you need to remove the existing index of that element as well. As array in java is immutable so you need to re-assign the array values to new array as you are doing already with newArr[index] Just to remove array elements without altering index you may use the predefined library of commons.apache.org like this ...
java - How to remove element from an array - Stack Overflow
You cannot remove an element from an array. The size of a Java array is determined when the array is allocated, and cannot be changed. The best you can do is: Assign null to the array at the relevant position; e.g. test[1] = null; This leaves you with the problem of dealing with the "holes" in the array where the null values are. (In some cases ...
How to remove specific object from ArrayList in Java?
Dec 15, 2011 · If you want to remove multiple objects that are matching to the property try this. I have used following code to remove element from object array it helped me. In general an object can be removed in two ways from an ArrayList (or generally any List), by index (remove(int)) and by object (remove(Object)).
How do I remove a specific element from a JSONArray?
Jan 11, 2012 · To Remove some element from Listview in android then it will remove your specific element and Bind it to listview. BookinhHistory_adapter.this.productPojoList.remove(position); BookinhHistory_adapter.this.notifyDataSetChanged();