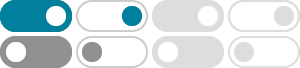
Java program to print triangle or reverse triangle using any …
Jun 14, 2021 · In this tutorial, we will show you how to print a Triangle in Java. We can print the Triangle using any character like *,&,$ etc.
beginner java - printing a right triangle backwards
"Write a program that will draw a right triangle of 100 lines in the following shape: The first line, print 100 '', the second line, 99 '’... the last line, only one '*'. Using for loop for this problem. Name the program as PrintTriangle.java" ***** **** *** ** *
Printing Reverse Triangle with Numbers - Java - Stack Overflow
Sep 24, 2014 · You just need to reverse the order of your loops and decrement x instead of incrementing it. I change the code a bit though : int level = 3; for(int r=level ; r>0 ; r--) { for(int c=r ; c>0 ; c--) for (int x=0 ; x<c ; x++) System.out.print("*"); System.out.println(); }
how to print reverse number pattern triangle in java
Mar 31, 2017 · Let the first loop (i) run from 1 to 4 and the second (j) from 4 to i. This reverses your output. You did every thing right, just the last for should have a very minor change: Here you go: for (int i = 1; i <= 4; i++) { for (int j = 4; j > i; j--) { System.out.print(" "); for (int k = i; k >= 1; k--){ System.out.print(k + ""); System.out.println();
4 ways in Java to print an inverted right-angled triangle
Jun 15, 2022 · Let’s learn how to print an inverted right-angled triangle in Java. We will write one program that will take the height of the triangle as an input from the user and print the inverted right-angled triangle using character like * , ^ etc.
Java Program to Print an Inverted Right Triangle Star Pattern
This Java program prints an inverted right triangle using stars (*). The number of stars decreases row by row, creating the inverted shape. This exercise is a useful way to practice working with loops and pattern generation in Java.
Java Program to Print Reverse Pyramid Star Pattern
Mar 20, 2023 · Methods: We can print a reverse pyramid star pattern using the following methods: Using while loop; Using for loop; Using do-while loop; Example 1: Using While Loop
Printing Triangle Pattern in Java - GeeksforGeeks
Mar 13, 2023 · Given the number N, the task is to print a pattern such that in each line all the digits from N to 1 are present in decreasing order and the frequency of the elements in ith line is N-i (the lines are 0 based i.e., i varies in the range [0, N-1]).
Java Program to Print Triangle of Alphabets in Reverse Pattern
Write a Java program to print triangle of alphabets in reverse pattern using for loop. private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); . System.out.print("Enter Triangle of Alphabets in Reverse Rows = "); int rows = sc.nextInt(); System.out.println("Printing Triangle of Alphabets in Reverse Order");
Java Program to Print Invert Triangle - W3Schools
This Java program is used to print Invert Triangle. Example: public class JavaInvertTriangle { public static void main(String args[]) { int num = 9; while(num > 0) { for(int i=1; i<=num; i++) { System.out.print(" "+num+" "); } System.out.print("\n"); num--; } } }