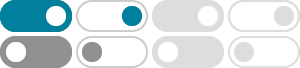
python - How to count the frequency of the elements in an …
You can use the in-built function provided in python. l.count(l[i]) d=[] for i in range(len(l)): if l[i] not in d: d.append(l[i]) print(l.count(l[i]) The above code automatically removes duplicates in a list and also prints the frequency of each element in original list and the list without duplicates.
Find frequency of each word in a string in Python
Feb 20, 2023 · Iterate over the set and use count function (i.e. string.count (newstring [iteration])) to find the frequency of word at each iteration. Implementation: Approach 3 (Using Dictionary): if elements[-1] == '.': Approach 4: Using Counter () function: Time Complexity: O (n)
Python – List Frequency of Elements - GeeksforGeeks
Feb 10, 2025 · Python - Fractional Frequency of elements in List Given a List, get fractional frequency of each element at each position. Input : test_list = [4, 5, 4, 6, 7, 5, 4, 5, 4] Output : ['1/4', '1/3', '2/4', '1/1', '1/1', '2/3', '3/4', '3/3', '4/4'] Explanation : 4 occurs 1/4th of total occurrences till 1st index, and so on.Input : test_list = [4, 5, 4,
Counting the Frequencies in a List Using Dictionary in Python
Feb 10, 2025 · Counting the frequencies of items in a list using a dictionary in Python can be done in several ways. For beginners, manually using a basic dictionary is a good starting point. It uses a basic dictionary to store the counts, and the logic …
Item frequency count in Python - Stack Overflow
May 21, 2009 · Assume I have a list of words, and I want to find the number of times each word appears in that list. An obvious way to do this is: words = "apple banana apple strawberry banana lemon" uniques = set(words.split()) freqs = [(item, words.split().count(item)) for …
Count the Frequency of Elements in a List
Aug 20, 2021 · We can count the frequency of elements in a list using a python dictionary. To perform this operation, we will create a dictionary that will contain unique elements from the input list as keys and their count as values.
python - Count frequency of words in a list and sort by frequency ...
Here's the basic design: original list = ["the", "car",....] newlst = [] frequency = [] for word in the original list. if word not in newlst: newlst.append(word) set frequency = 1. else. increase the frequency. sort newlst based on frequency list . it's hard for us to know what you know.
Python: Count the frequency of each word in a string (2 ways)
May 27, 2023 · This succinct and straight-to-the-point article will walk you through some different ways to find the frequency (the number of occurrences) of each word in a string in Python. This approach is very concise since it utilizes the Counter class from the collections module. The steps are: Import the Counter class from the collections module.
3 Ways to Count the Item Frequencies in a Python List
Nov 2, 2022 · To get the frequency of all items at once, we can use one of the following two ways. The counter function in the collections module can be used for finding the frequencies of items in a list. It is a dictionary subclass and used for counting hashable objects.
How to Count the Frequency of Elements in a Python List? - Python …
Mar 4, 2025 · Learn how to count the frequency of elements in a Python list using `Counter` from `collections`, `dict.get()`, and loops. This guide includes examples.