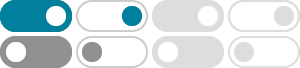
Java Program to Remove Duplicate Elements From the Array
Nov 16, 2024 · Given an array, the task is to remove the duplicate elements from an array. The simplest method to remove duplicates from an array is using a Set , which automatically eliminates duplicates. This method can be used even if the array is not sorted.
How to Efficiently Remove Duplicates from an Array
Apr 27, 2024 · Removing duplicate elements from an array is a common operation that can be easily accomplished using sets. However, in this article, we will learn how to remove duplicates from an array in Java without using a set, in an efficient manner.
Delete duplicate elements from an array - Stack Overflow
Try following from Removing duplicates from an Array(simple): Array.prototype.removeDuplicates = function (){ var temp=new Array(); this.sort(); for(i=0;i<this.length;i++){ if(this[i]==this[i+1]) {continue} temp[temp.length]=this[i]; } return temp; }
algorithm - Array remove duplicate elements - Stack Overflow
Jul 28, 2010 · Put all entries (in the unique sorted array) into a hashtable, which has O (1) access. Then iterate over the original array. For each element, check if it is in the hash table. If it is, add it to the result and delete it from the hash table.
How can I remove duplicates from array without using another array?
May 17, 2020 · You can do this by simply overwriting the elements at the front of the array and keeping track of the count. At the end, the solution will be the front slice of the array from 0 to count: This will be linear time and constant space. maybe add a …
5 Effective Methods in Java to Remove Array Duplicates
Dec 12, 2023 · We can leverage Streams to remove duplicates from an unsorted array while maintaining the order of elements. To begin, we need to convert our array into a Stream using the Arrays.stream() method. This allows us to perform various operations on …
C program to delete duplicate elements from array - Codeforwin
Jul 12, 2015 · How to remove duplicate elements from array in C programming. After performing delete operation the array should only contain unique integer value. Logic to delete duplicate elements from array. Basic Input Output, If else, For loop, Nested loop, Array. Step by step descriptive logic to delete duplicate elements from array.
Remove Duplicate Elements From Array
Jan 18, 2023 · Remove Duplicate elements from array is a relatively straightforward process. In this blog post, our focus will be on eliminating duplicate elements from an array. We will explore methods to identify and efficiently remove these duplicates, streamlining the array for improved clarity and efficiency.
Mastering Array Manipulation: Removing Duplicates and Finding …
Oct 13, 2024 · Given a sorted array of integers , remove duplicate in-place such that each unique element appears only once. The relative order of the elements should be preserved. example: Input...
How to Remove Duplicates from Arrays - Medium
Mar 1, 2021 · The filter method can be used to removed duplicates from an array together with the indexOf and findIndex methods.