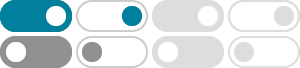
python - Convert elements of a list into binary - Stack Overflow
How can I make python interpret this list as a binary number 0100 so that 2*(0100) gives me 01000? The only way that I can think of is to first make a function that converts the "binary" elements to corresponding integers (to base 10) and then use bin () function..
5 Best Ways to Convert Python Lists to Binary - Finxter
Feb 20, 2024 · Python’s built-in function bin() converts an integer to its binary string equivalent. Combining bin() with list comprehension provides a straightforward method to convert each integer in the list to binary format. This method is both readable and efficient for those familiar with list comprehension. Here’s an example: Output:
Python | Decimal to binary list conversion - GeeksforGeeks
Apr 21, 2023 · to convert a decimal number to a binary list in Python is to use bit manipulation operations to extract the binary digits from the decimal number and append them to a list.
Unveiling the Magic of `bin ()` in Python - CodeRivers
3 days ago · In the world of Python programming, the `bin ()` function is a valuable tool when dealing with numbers in binary representation. Binary numbers are the foundation of how computers store and process data at the most fundamental level. Understanding how to convert numbers to binary and work with the results using `bin ()` can be extremely useful in various applications, such as low - level ...
convert list to binary python - Code Examples & Solutions
0 List = [0, 1, 0, 1, 0, 1] print ("The List is : " + str (List)) # binary list to integer conversion result = int ("".join (str (i) for i in List),2) # result print ("The value is : " + str (result))
Converting integer to binary in Python - Stack Overflow
To convert a binary string back to integer, use int () function. returns integer value of binary string. Better would be format (a, '08b') to obtain the format the user wanted. .. or if you're not sure it should always be 8 digits, you can pass it as a parameter: Going Old School always works. while (number>=1): if (number %2==0):
How to convert numbers to binary in Python - dhairyashah.dev
Sep 25, 2023 · Python offers a simple and straightforward way to convert decimal numbers to binary using the built-in bin() function. Here’s how you would use it: The bin() function takes a …
Top 6 Methods to Write a List of Numbers as Bytes to a Binary File
Nov 6, 2024 · One effective approach is to use the struct.pack function, which converts Python values into a binary format. Here’s a typical implementation: newFileBytes = [120, 3, 255, 0, 100] with open("output.bin", "wb") as newFile: newFile.write(struct.pack('5B', *newFileBytes))
Convert Integer to Binary List and Find First 1 Position in Python
In Python, you can write a function that converts an integer into a binary number represented as a list. The function should read the binary digits from left to right and return the position of the first 1 in the binary representation. This can be achieved without using the bin, %, or // operators. Here's an example implementation of such a ...
Converting Integers to Binary in Python: A Comprehensive Guide
Feb 18, 2025 · The bin() function in Python is the most straightforward way to convert an integer to its binary representation. The syntax is bin(integer), where integer is the integer value you want to convert.
- Some results have been removed