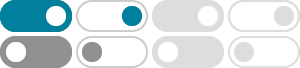
c# - Searching a tree using LINQ - Stack Overflow
If you wanted a (first-child-first) breadth-first search, you can change the type of the nodes collection to Queue<Node> (with the corresponding changes to Enqueue/Dequeue from Push/Pop).
Generic Linear Search/Sequential Search for a sequence in C# …
Aug 6, 2021 · To practice algorithms and data structures, I reimplemented Linear Search/Sequential Search for a sequence using C#'s generic type parameters.
Recursively traversing a tree in C# from top down by row
Aug 19, 2010 · How would you go about traversing down the tree from the root node, by row such that you find the first node with a specific value. So say that each node has 3 attributes: its name/location, the identity of its parent, and who "owns" the node.
C# Sequential Search Example | C# Examples
Sequential search (Linear search) is the simplest search algorithm. It is a special case of brute-force search. It is a method for finding a particular value in a list. To achieve this, it checks every one of its elements one. Usage: //Sorted array int [] arr = new int [10] { 1, 2, 4, 11, 20, 28, 48,
C# Binary Search Tree Implementation | C# Examples
May 22, 2020 · This example shows how to implement a Binary Search Tree using C#. A tree whose nodes have at most 2 child nodes is called a binary tree. we name them the left and right child because each node in a binary tree can have only 2 children. A sample binary tree: Tree Traversals (PreOrder, InOrder, PostOrder)
algorithm - Traversing a tree of objects in c# - Stack Overflow
Jul 11, 2016 · Keep it simple using Recursion. An algorithm which uses recursion goes like this: printTitle(node.title) foreach (Node child in node.children) printNode(child); //<-- recursive.
Searching Algorithms In C# - C# Corner
In this blog, I am going to discuss two of the most commonly-used searching algorithms in the programming world - I will be explaining the algorithms with the help of an example and will provide a C# code to execute that. Linear Search. This algorithm will perform a sequential search of item in the given array.
Searching in C#.Net, Types of Searching, Their Implementations
Dec 15, 2011 · In this tutorial, we will learn about C# .Net searching, its types (Linear/Sequential and Binary) and implementation. Here, you will also find the solved programs on searching in C#.Net.
Recursive search on Node Tree with Linq and Queue
Jul 22, 2019 · I fixed the following: A) IsRoot code (now, if the parent is not set, then it's considered as the root ; B) Removed the null check on Children; C) Search method now returns immutable copies of the descendants
Data Structures and Algorithms (DSA) using C# .NET Core — Binary Trees …
Dec 31, 2023 · In linear data structures like linked list, arrays, stacks and queues, the data is stored in sequential manner and there is only one way to read the data, but in case of Trees the data is...