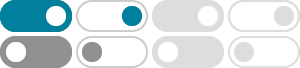
python - Is there a numpy function that allows you to specify start ...
return np.fromiter(itertools.count(start, step), np.float, num) ... >>> my_range(0, 0.1, 3) array([ 0. , 0.1, 0.2]) You could make the np.float an arg (or kwarg) if you want to use it with something other than floats: >>> import numpy as np >>> import itertools >>> def my_range(start, step, num, dtype=np.float): ...
numpy.arange — NumPy v2.2 Manual
numpy. arange ([start, ] stop, [step, ] dtype=None, *, device=None, like=None) # Return evenly spaced values within a given interval. arange can be called with a varying number of positional arguments:
Range with Steps - Python Examples
Learn how to create a range in Python with a specified step value using the range () function. This tutorial covers the syntax and provides clear examples, demonstrating how to iterate through sequences with customizable step increments.
slice - How slicing in Python works - Stack Overflow
To skip specifying a given argument, one might use None, so that e.g. a[start:] is equivalent to a[slice(start, None)] or a[::-1] is equivalent to a[slice(None, None, -1)]. While the : -based notation is very helpful for simple slicing, the explicit use of slice() objects simplifies the programmatic generation of slicing.
Allow `range (start, None, step)` for an endless range
Jan 15, 2021 · I propose using range (start, None, step) for an endless range. This makes sense, as the ‘stop’ parameter is None, i.e. there is no ‘stop’ and the iterable goes on forever. The step and start parameters could be omitted as normal. I would also suggest range () should default to an end of None with zero arguments: Downsides:
What does "step" mean in the range function? | Codecademy
The step is each increment it goes up by. For instance, if you had range( 0, 100, 2 ) it would be 0,2,4,6,8,10,… In the example range(1,6,3) is would print out 1 and 4. The next step in the range is 7 but the stop doesn’t go that high.
Python range() Function Explained with Examples - PYnative
Mar 17, 2022 · Python range() function generates the immutable sequence of numbers starting from the given start integer to the stop integer. The range() is a built-in function that returns a range object that consists series of integer numbers, which we can iterate using a for loop.
python - No step set via 'step' argument or …
May 22, 2020 · Try passing the epoch using tf.summary.scalar(name, value, step=epoch). You obtain this value when def on_epoch_end(self, epoch, logs=None) is called, but you're not passing it to following functions.
Start Stop Step Python | slice() Parameters - EyeHunts
Aug 12, 2021 · Here is a Python example of the slice method with the Start, Stop, and Step Arguments (Parameters). If the only stop is provided, it generates a portion of sequence from index 0 till stop a = [1, 2, 3, 4, 5, 6, 7, 8] print(a[:5])
AttributeError: ‘numpy.ndarray’ object has no attribute ‘step’ in Python
The error message "AttributeError: ‘numpy.ndarray’ object has no attribute ‘step’" in Python indicates that you are trying to access the ‘step’ attribute of a NumPy ndarray, but this attribute does not exist for ndarrays.