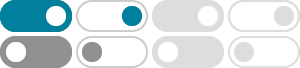
LIMITATION Definition & Meaning - Merriam-Webster
The meaning of LIMITATION is an act or instance of limiting. How to use limitation in a sentence.
LIMITATION | English meaning - Cambridge Dictionary
Living in an apartment is fine, but it does have its limitations - for example, you don't have your own garden. [ C ] The major limitation of early record players was the short playing time of the …
LIMITATION Definition & Meaning | Dictionary.com
Limitation definition: a limiting limiting condition; restrictive weakness; lack of capacity; inability or handicap.. See examples of LIMITATION used in a sentence.
LIMITATION definition and meaning | Collins English Dictionary
If you talk about the limitations of someone or something, you mean that they can only do some things and not others, or cannot do something very well. The theory is a useful tool, but it has …
limitation noun - Definition, pictures, pronunciation and usage …
Definition of limitation noun from the Oxford Advanced Learner's Dictionary. [uncountable] the act or process of limiting or controlling somebody/something synonym restriction. They would …
Limitation - Definition, Meaning & Synonyms | Vocabulary.com
A limitation is something that holds you back, like a broken leg that keeps you off the dance floor during prom season. A limitation could also be a rule that restricts what you can do, like …
Limitations - definition of limitations by The Free Dictionary
1. a limiting condition; restrictive weakness; lack of capacity: to know one's limitations. 2. something that limits; a limit or bound; restriction. 3. the act of limiting. 4. the state of being …
LIMITATION Synonyms: 52 Similar and Opposite Words - Merriam-Webster
Recent Examples of Synonyms for limitation. The Senate bill would reduce the deductions for individuals making over $150,000, while the House bill does not include income limits. And …
Limitation Definition & Meaning | Britannica Dictionary
They have placed a limitation on the amount of time we have available. There are strict limitations on the uses of these funds. We'd like to include more material, but space limitations make that …
LIMITATIONS | English meaning - Cambridge Dictionary
LIMITATIONS definition: 1. If someone or something has limitations, that person or thing is not as good as they or it could…. Learn more.